Hey everyone, it’s your boy back again with another one of my little projects. Today, I wanted to mess around with keeping track of points in a volleyball game. I’ve always been a fan of the sport, but I never really dug into the nitty-gritty of the scoring system. So, I thought, why not build something to help me understand it better?
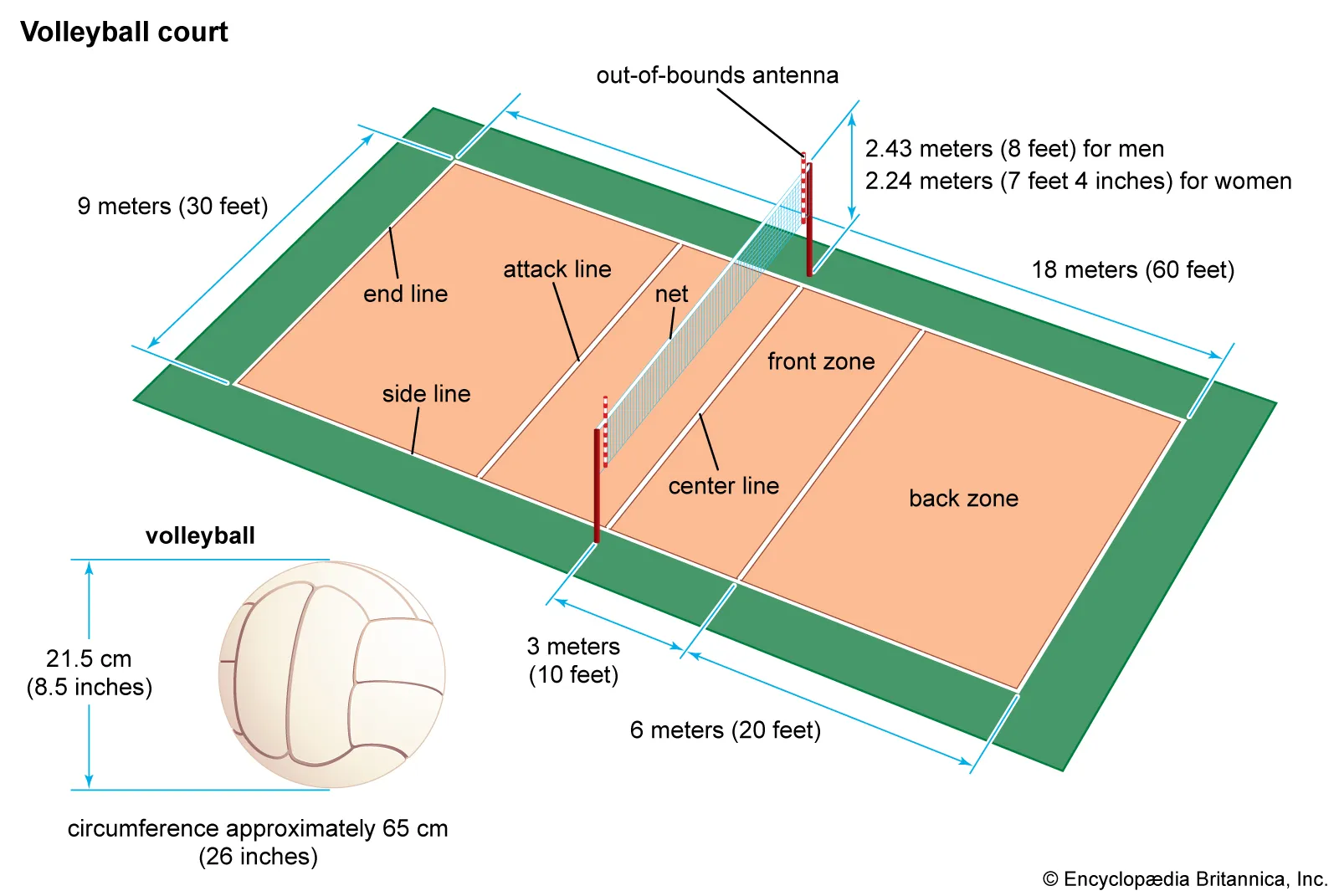
First things first, I had to figure out how the scoring actually works. I mean, I knew the basics – you gotta get to 25 points, and if it’s a tiebreaker, it’s 15. But there’s also that whole “win by two” thing. So, I did a little digging around online, just to make sure I had it straight.
After I felt like I had a good grasp on the rules, I started brainstorming how I wanted to approach this. I decided to make a simple program that would let me input the scores for each team and then calculate the winner of each set, and eventually, the whole match.
I started by outlining the basic structure. I knew I’d need variables to store the scores for each team, and some way to keep track of which set we were on. I also figured I’d need some conditional statements to check if a team had reached 25 points (or 15 in the fifth set) and if they were ahead by at least two.
Next, I got down to coding. I chose to use Python because, well, it’s just what I’m most comfortable with. I started by setting up my variables and getting the initial input from the user for the team names.
Here’s a snippet of what that looked like:
team1_name = input("Enter the name of team 1: ")
team2_name = input("Enter the name of team 2: ")
team1_score = 0
team2_score = 0
set_number = 1
Then came the fun part – the main game loop. I used a while
loop to keep the game going until one team had won three sets. Inside the loop, I had another loop that ran until one team won the current set.
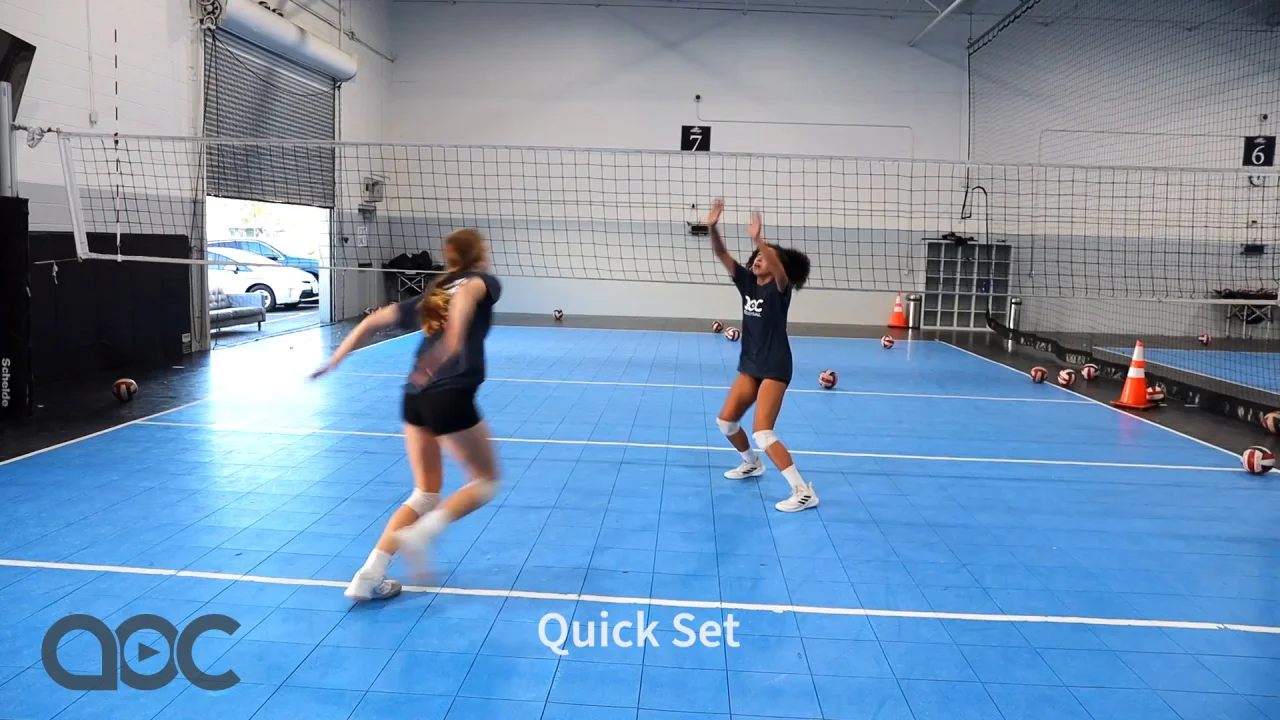
Inside the set loop, I prompted the user to enter the scores for each team after each point. Then, I checked if either team had met the conditions to win the set.
Here’s how that logic looked:
while (team1_score < 25 and team2_score < 25) or abs(team1_score - team2_score) < 2:
# Get scores...
# Check if either team has won by 2
If a team won the set, I updated the set number, reset the scores to 0, and printed out who won the set. I kept this up until one team had won three sets, and then I declared them the winner of the match.
It was pretty satisfying to see it all come together. I ran a bunch of test games, inputting different scores to make sure it was handling the “win by two” rule correctly, and it seemed to be working like a charm.
The End
All in all, this was a fun little project. Not only did I get to practice my coding skills, but I also got a better understanding of how volleyball scoring works. And hey, maybe I’ll use this program to keep score at my next volleyball party. Who knows? Anyway, hope you found this interesting. Catch you in the next one!